Prerequisites
- JDK 1.5 and above
- Jfreechart jar
- mysql connector jar
To generate charts we can use jfrecharts which is open source and free. In this example i will show how to generate the jfreechart dynamically for every 5 seconds getting data from the database.
Full source code : github link
sample :
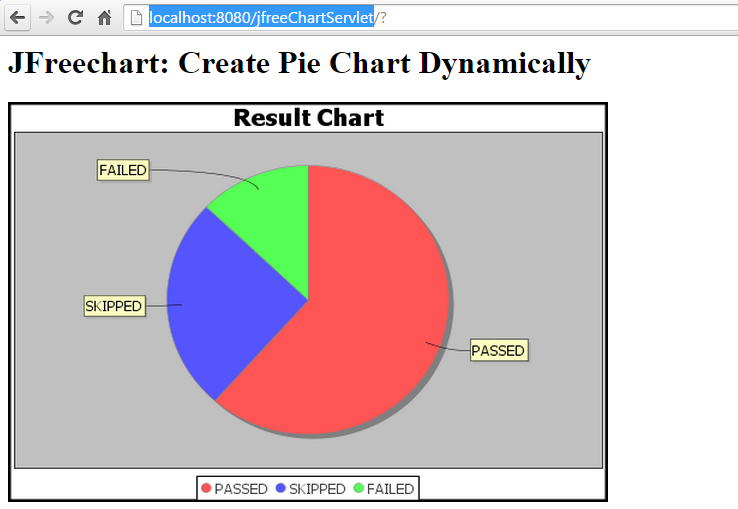
Create Simple servlet :
import java.awt.BasicStroke; import java.awt.Color; import java.io.IOException; import java.io.OutputStream; import java.sql.*; import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.util.ArrayList; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.jfree.chart.ChartFactory; import org.jfree.chart.ChartRenderingInfo; import org.jfree.chart.ChartUtilities; import org.jfree.chart.JFreeChart; import org.jfree.chart.entity.StandardEntityCollection; import org.jfree.data.jdbc.JDBCPieDataset; public class JfreeChartServlet extends HttpServlet { private static Connection connection = null; protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { try { JDBCPieDataset dataset = new JDBCPieDataset(getConnection()); dataset.executeQuery("select * from result"); JFreeChart chart = ChartFactory.createPieChart("Result Chart", dataset, true, true, false); chart.setBorderPaint(Color.black); chart.setBorderStroke(new BasicStroke(4.0f)); chart.setBorderVisible(true); if (chart != null) { int width = 500; int height = 350; final ChartRenderingInfo info = new ChartRenderingInfo(new StandardEntityCollection()); response.setContentType("image/png"); OutputStream out = response.getOutputStream(); ChartUtilities.writeChartAsPNG(out, chart, width, height, info); } } catch (SQLException e) { e.printStackTrace(); } } public static Connection getConnection() { if (connection != null) return connection; else { try { String driver = "com.mysql.jdbc.Driver"; String url = "jdbc:mysql://localhost:3306/test"; String user = "root"; String password = "******"; Class.forName(driver); connection = DriverManager.getConnection(url, user, password); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (SQLException e) { e.printStackTrace(); } return connection; } } public static ArrayList<Result> getData() { connection = getConnection(); ArrayList<Result> resultList = new ArrayList<Result>(); try { Statement statement = connection.createStatement(); ResultSet rs = statement.executeQuery("select * from result"); while(rs.next()) { Result result=new Result(); result.setStatus(rs.getString("status")); result.setCount(rs.getInt("count")); resultList.add(result); } } catch (SQLException e) { e.printStackTrace(); } return resultList; } }
jfreechartSample.jsp :
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Pie Chart Demo</title> <script language="Javascript"> function refreshpage(){ document.forms.form1.submit(); } </script> </head> <body> <h1>Pie Chart</h1> <%response.setIntHeader("Refresh",5);%> <form id="form1"> <img src="chart" width="600" height="400" border="0"/> <!-- <input type="button" onclick="refreshpage()" value="Refresh"/>--> </form> </body> </html>
web.xml:
<?xml version="1.0" encoding="UTF-8"?> <web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"> <servlet> <servlet-name>JfreeChartServlet</servlet-name> <servlet-class>JfreeChartServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>JfreeChartServlet</servlet-name> <url-pattern>/chart</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>jfreechartSample.jsp</welcome-file> </welcome-file-list> </web-app>
Database scripts: create the following table and insert the sample data.
CREATE TABLE `result` ( `status` varchar(20) DEFAULT NULL, `count` int(11) DEFAULT NULL ); INSERT INTO result( status ,count ) VALUES ( 'PASSED' -- status - IN varchar(20) ,25 -- count - IN int(11) ); INSERT INTO result( status ,count ) VALUES ( 'FAILED' -- status - IN varchar(20) ,40 -- count - IN int(11) ); INSERT INTO result( status ,count ) VALUES ( 'SKIPPED' -- status - IN varchar(20) ,10 -- count - IN int(11) )
Jchart Servelt Example ~ Uttesh Tech Blogs >>>>> Download Now
ReplyDelete>>>>> Download Full
Jchart Servelt Example ~ Uttesh Tech Blogs >>>>> Download LINK
>>>>> Download Now
Jchart Servelt Example ~ Uttesh Tech Blogs >>>>> Download Full
>>>>> Download LINK lt