What is Selenium Grid?
Selenium Grid is a part of the Selenium Suite that specializes on running multiple tests across different browsers, operating systems, and machines in parallel.
With the release of Selenium 2.0, the Selenium Server now has built-in Grid functionality.
The selenium-server-standalone package includes the Hub, WebDriver, and legacy RC needed to run the grid. Ant is not required anymore!!!!
Selenium Grid uses a hub-node concept, where test cases will be running on a single machine called a hub, but the execution will be done by different machines called nodes. i.e node will have the browser drivers and hub will passing your test cases to each node and that will be executing.
Why we have to use Selenium Grid?
- Run your tests against different browsers/operating systems and machines all at the same time.This will ensure that the application you are testing is fully compatible with a wide range of browser-OS combinations.
- Save time in execution of your test suites. If you set up Selenium Grid to run, say, 4 tests at a time, then you would be able to finish the whole suite around 4 times faster.
The Hub
- The hub is the central point where you load your tests into.
- There should only be one hub in a grid.
- The hub is launched only on a single machine, say, a computer whose OS is Windows XP/7/vista/8 and whose browser is IE.
- The machine containing the hub is where the tests will be run, but you will see the browser being automated on the node.
- Nodes are the Selenium instances that will execute the tests that you loaded on the hub.
- There can be one or more nodes in a grid.
- Nodes can be launched on multiple machines with different platforms and browsers.
- The machines running the nodes need not be the same platform as that of the hub.
example : HUB : Machine H NODE1 : Machine IE(which as IE driver) NODE2 : Machine CHROME(which as CHROME driver) NODE3 : Machine FIREFOX (which as FIREFOX driver)
How to configure the selenium server for remote/virtual machine web drivers?
Quick Start:
1.Download the respective webdriver and keep in proper location.
2.Download the selenium server/client jar files.
3. run the selenium server jar which will act as hub as mentioned below
java -jar selenium-server-standalone-2.37.0.jar -role hub
this hub will act as center point for access for all remote web driver pull and it will monitor all nodes.
http://localhost:4444/grid/console
4. run the selenium server jar as node which will provite webdrivers
change localhost to ip address if hub is running in different box/vm.
java -jar selenium-server-standalone-2.37.0.jar -role node -hub http://localhost:4444/grid/register
-browser browserName="internet explorer",
version=11,
maxInstances=1,
platform=WINDOWS
-Dwebdriver.ie.driver=
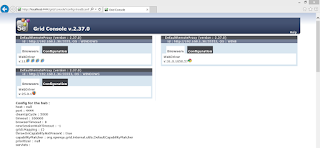
5. Running test from grid
Selenium selenium = new DefaultSelenium(“localhost”, 4444, “*firefox”, “http://www.uttesh.blogspot.com”);
We should use remote driver with desiredCapabilities object to define which browser, version and platform you wish to use.
DesiredCapabilities capability = DesiredCapabilities.firefox();
RemoteWebDriver object
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capability);
A node matches if all the requested capabilities are met. To request specific capabilities on the grid, specify them before passing it into the WebDriver object
i.e.
capability.setBrowserName(); capability.setPlatform(); capability.setVersion() capability.setCapability(,);
6. Now both hub and node are running, we have to implement the code to get the driver from hub.
for full sample click here
to get the full selenium simple junit sample go to my github https://github.com/uttesh/SeleniumGridJavaAnt
Summary
- Selenium Grid is used to run multiple tests simultaneously in different browsers and platforms.
- Grid uses the hub-node concept.
- The hub is the central point wherein you load your tests.
- Nodes are the Selenium instances that will execute the tests that you loaded on the hub.
- There are 2 ways to verify if the hub is running: one was through the command prompt, and the other was through a browser
- To run test scripts on the Grid, you should use the DesiredCapabilities and the RemoteWebDriver objects.
- DesiredCapabilites is used to set the type of browser and OS that we will automate
- RemoteWebDriver is used to set which node (or machine) that our test will run against.